A reader recently asked how he could register an Exchange 2000/2003/2007 EventSink so that it would only fire for items with these properties:
- Not hidden
- An appointment item.
- The appointment is not private/personal/confidential.
- The appointment is labeled blue/business/label (this is stored on property http://schemas.microsoft.com/mapi/id/{00062002-0000-0000-C000-000000000046}/0x8214 which is of type int. The required value is 2).
He tried registering the EventSink with the following restriction for the RegEvent.vbs script:
"WHERE $DAV:ishidden$ = FALSE AND $DAV:contentclass$ = 'urn:content-classes:appointment' AND $http://schemas.microsoft.com/mapi/sensitivity$ = 0 AND $http://schemas.microsoft.com/mapi/id/{00062002-0000-0000-C000-000000000046}/0x8214$ = 2"
This didn’t work. The problem is that Exchange is not very smart about guessing the types of properties which are not in it’s schema. It treats them all as strings. So, while a simple comparison is sufficient for the sensivity property, the appointment color property must be cast to int.
The correct where clause is this:
"WHERE $DAV:ishidden$ = FALSE AND $DAV:contentclass$ = 'urn:content-classes:appointment' AND $http://schemas.microsoft.com/mapi/sensitivity$ = 0 AND Cast($http://schemas.microsoft.com/mapi/id/{00062002-0000-0000-C000-000000000046}/0x8214$ as int) = 2"
2883cc93-a5ff-4138-a2fe-4336eaaa8a6c|0|.0
Although Eventsinks are being removed from the next version of Exchange (see Exchange Developer Roadmap - WebDAV and StoreEvents gone), they are widely used and sometimes the only option. Especially when working with tasks, contacts and appointments.
But writing Eventsinks has always been a pain because all this interop stuff, COM+ registration issues and more. Attached to this post you can find a small framework which simplifies writing event sinks (both synchronous and asynchronous ones).
The InfiniTec.Exchange.Eventing assembly contains several base classes from which you inherit your Eventsink. The only thing you have to do is to overwrite some methods (OnItemChanged, OnItemCreated, OnItemCreated) and you get relevant information about the event which happened in a compact object (EventInfo class). Below is a class diagram of the InfiniTec.Exchange.Eventing namespace:
Depending whether you want to create an asynchronous or synchronous Eventsink, you derive your sink from either SynchronousEventSink or AsynchronousEventSink. In you derived class, you simply override the notifications you want to catch. One thing all Eventsinks have in common is the registration process – Implemented by the OnRegisteringEvent. By returning false from this method you can prevent the registration on a certain url.
The synchronous Eventsink is called two times for each event: The first execution is called the Begin Phase; during this stage, the item is writable and you can even check for changes by loading the original item (via EventInfo.OpenItemLocally()). The seconds stage is called Commit Phase; the item is read-only now. If you need to share information about a particular event between these to stages, set the EventInfo.UserState property. The object graph is serialized using the BinarySerializer during the begin phase, so each instance used here must be serializable. This is a feature which was not possible with the interop files generated with tlbimp, because that tool did not generate the proper interop code. Since I’ve incorporated the complete interop code in this assembly, I fixed the signature of the affected interface.
Correctly deciphering the flags passed to the Evensink was an art of it’s own, so I’ve cleaned up those flags enumerations as well and routed them different methods (OnItemCreated with its CreationMode parameter and OnItemDeleted with its DeletionMode parameter).
At last, you don’t need the the regevent.vbs script any longer because I’ve included an EventSinkInstaller which registers your EventSink on a certain folder.
Here is a sample event sink:
1: using System;
2: using System.Runtime.InteropServices;
3: using ADODB;
4: using InfiniTec.Exchange.Eventing;
5:
6: namespace TestSink
7: {
8: [ComVisible(true)]
9: [Guid("FD0D03A3-9FD2-432b-B331-E7C4D412827F")]
10: [ProgId("TestSink.TestSink")]
11: public class TestSink: SynchronousEventSink
12: {
13: protected override void OnItemCreating(EventInfo info, CreationMode creationMode)
14: {
15: // Do something of interest here - you have read/write access to the item
16: Record item = info.Item;
17:
18: // you can save an object here (it must be serializable) and reuse it during the OnItemCreated method
19: info.UserState = "test";
20:
21:
22: base.OnItemCreating(info, creationMode);
23: }
24:
25: protected override void OnItemCreated(EventInfo info, CreationMode creationMode)
26: {
27: base.OnItemCreated(info, creationMode);
28:
29: // Do something of interest here - the item is readonly now.
30:
31: // will be "test"
32: string s = (string) info.UserState;
33: }
34:
35:
36: protected override void OnItemDeleted(EventInfo info, DeletionMode deletionMode)
37: {
38: base.OnItemDeleted(info, deletionMode);
39: }
40:
41: protected override void OnItemUpdated(EventInfo info)
42: {
43: base.OnItemUpdated(info);
44: }
45:
46: protected override void OnErrorOccured(EventInfo eventInfo, Exception ex)
47: {
48: base.OnErrorOccured(eventInfo, ex);
49: }
50:
51: protected override void OnInitialize()
52: {
53: base.OnInitialize();
54: }
55:
56: protected override bool OnRegisteringEvent(EventInfo info)
57: {
58: return base.OnRegisteringEvent(info);
59: }
60:
61: protected override void OnItemCreationAborted(EventInfo info, CreationMode creationMode)
62: {
63: base.OnItemCreationAborted(info, creationMode);
64: }
65:
66: protected override void OnItemDeleting(EventInfo info, DeletionMode deletionMode)
67: {
68: base.OnItemDeleting(info, deletionMode);
69: }
70:
71: protected override void OnItemDeletionAborted(EventInfo info, DeletionMode deletionMode)
72: {
73: base.OnItemDeletionAborted(info, deletionMode);
74: }
75:
76: protected override void OnItemSilentlySaving(EventInfo info)
77: {
78: base.OnItemSilentlySaving(info);
79: }
80:
81: protected override void OnItemUpdateAborted(EventInfo info)
82: {
83: base.OnItemUpdateAborted(info);
84: }
85:
86: protected override void OnItemUpdating(EventInfo info)
87: {
88: base.OnItemUpdating(info);
89: }
90: }
91: }
As I did here, you should always add those three attributes to the class, albeit with different values for the Guid and ProgId attribute. And you should add these lines to your assemblyinfo file:
1: [assembly: ApplicationActivation(ActivationOption.Server)]
2: [assembly: ApplicationName("TestSink")]
3: [assembly: ApplicationAccessControl(false, AccessChecksLevel = AccessChecksLevelOption.Application)]
Be sure to customize the ApplicationName attribute, though.
Now that you have created an Eventsink, you should add an installer so you can deploy the solution more easily:
1: using System.Collections;
2: using System.ComponentModel;
3: using System.Configuration.Install;
4: using InfiniTec.Exchange.Eventing;
5:
6: namespace TestSink
7: {
8: [RunInstaller(true)]
9: public class TestInstaller: Installer
10: {
11: public override void Commit(IDictionary savedState)
12: {
13: Initialize();
14: base.Commit(savedState);
15: }
16:
17: public override void Install(IDictionary stateSaver)
18: {
19: Initialize();
20: base.Install(stateSaver);
21: }
22:
23: public override void Rollback(IDictionary savedState)
24: {
25: Initialize();
26: base.Rollback(savedState);
27: }
28:
29: public override void Uninstall(IDictionary savedState)
30: {
31: Initialize();
32:
33: base.Uninstall(savedState);
34: }
35:
36: private void Initialize()
37: {
38: var url = Context.Parameters["url"] + "/eventsink.evt";
39:
40: Context.LogMessage("Binding Url: " + url);
41: string criteria = Context.Parameters["Criteria"] ?? string.Empty;
42: criteria = criteria.Replace("$", "\"");
43:
44: Installers.Add(new EventSinkInstaller<TestSink>
45: {
46: Criteria = criteria,
47: EventMethods = EventMethods.SynchronousEvents,
48: Scope = MatchScope.Shallow,
49: Url = url
50: });
51: }
52: }
53: }
The reason why the Initialize() method is called in each of the methods is that the installer requires the url to the path on which the sink is registered. Unfortunately, the Context property is set after the constructor is run. What you basically need to do is to add an EventSinkInstaller the list of installers executed. The EventSinkInstaller has a type parameter which you can use to specify the type of sink registered. The installer will use this type parameter to determine the correct ProgId for the Eventsink. This example registers a synchronous event with a MatchScope of Shallow on the folder specified via the “url” parameter. Additionally, the constraint for the Eventsink is taken from the “constraint” parameter. This approach is superior to the regevent.vbs script because it lets you as the developer decide which parameters are fixed (the scope and type of the EventSink in this case) and which are variable, making the whole registration purpose less prone to errors.
Debugging
The InfiniTec.Exchange.Eventing assembly also defines a TraceSource which you can use for debugging. The TestSink application attached to this article contains the following application.config to activate the trace component:
1: <?xml version="1.0" encoding="utf-8" ?>
2: <configuration>
3: <system.diagnostics>
4: <trace autoflush="true" />
5: <sources>
6: <source name="infinitec.exchange.eventing"
7: switchName="verbosity"
8: switchType="System.Diagnostics.SourceSwitch">
9: <listeners>
10: <add name="listener"
11: type="System.Diagnostics.TextWriterTraceListener"
12: initializeData="c:\temp\TextWriterOutput.log" />
13: </listeners>
14: </source>
15: </sources>
16: <switches>
17: <add name="verbosity" value="All"/>
18: </switches>
19: </system.diagnostics>
20: </configuration>
Licensing
You may use this code (InfiniTec.Exchange.Eventing and InfiniTec.Common) in your application, regardless whether it is personal or business, free of charge.
Downloads
529f5c48-dd97-4477-9aa0-c28b73adb1e8|2|4.5
Microsoft just released a post about the technologies being removed from the next version of Exchange. I'm ok with WebDAV being removed, given that EWS will be extended to support access to hidden messages and providing strong-typed access to mailbox settings. I can also live with the fact that CdoEx and ExOleDB will be remove. But store events are another thing.
Sure, they are not really easy to implement and the whole access using ADO is a terrible mess. CdoEx makes it not exactly better as there is no support for tasks.
The proposed replacement for store event sinks are transport agents and EWS notification. While transport agents are fine when dealing with email messages, they are useless with respect to appointments, contacts and tasks. This leaves the EWS notifications as the sole option here. Why is this bad?
- Synchronous execution (access to old data as well): Synchronous event sinks (OnSyncSave, OnSyncDelete) provide direct access to the item being modified (the record is directly available). And during the Begin phase, the event sink can even open the old record and execute actions based on how fields where changed. This feature will be lost completely with EWS notifications.
- Register once, even for new users (storewide eventsinks): Store wide event sinks are registered once on a store and it will be triggered for every mailbox in the store - even new users. EWS notifications must be registered for each mailbox and the application receiving the mails is required to monitor Exchange for new mailboxes.
- Access to all properties, even during deletion of an object: With a synchronous OnSyncDelete event sink, all properties of an item can be examined before it gets deleted. With notifications I merely get a notification that and item with a specific ItemId has been deleted. The client application is responsible to track the deleted item - whether is was soft-deleted or moved to the recycle bin. The properties can then be accessed from there. But if the item was hard-deleted (in case of the dumpster being disabled on public folders, for example), one is out of luck. The real problem is this: The ItemId is based on the email address of the mailbox owner as well as the MAPI EntryId of the item (see Exchange 2007 SP1 Item ids). Both, the mailbox address as well as the MAPI entry id are not guaranteed to be stable (Since Exchange 2003 SP2, Exchange recreates meeting items under certain circumstances: CDOEX Calendaring Differences Between Exchange 2003 and Exchange 2003 SP2). This has the effect that the ItemId is not suitable as a long term identifier which should be stored in a database. In scenarios where Exchange data is being replicated to a relational databases, this can become a problem.
- Order of execution: Synchronous event sinks are called in the order the items were changed. With asynchronous notifications, this cannot be guaranteed.
To clarify this: The order in which notifications are sent to the client application cannot guaranteed to reflect the order in which the changes were made. To work around this issue, the each notification carries a watermark and a previous watermark. This way a client application can restore the correct order. But with synchronous store events, this comes for free. - Silent updates: Due to the possibility to modify an item while it's being changed, the synchronous store events allow some sort of silent update. This works like this:
- Along with all the other modifications, a client program sets a special user defined field to an arbitrary value.
- The event sink checks this field during the Begin phase, and if it's set it won't process the item. Instead it just removes the property from the element.
- Modify changes made by a user / Block modifications: A synchronous event sink can modify changes while they are being saved. It can even abort the change, thus preventing a user from deleting an item for example.
Note: Canceling updates/deletions can break synchronization with ActiveSync or Outlook. So don't do this! - Performance: In most scenarios, the WebService receiving the push notifications will not reside on the Exchange server (I know a bunch of administrators who even hesitate to install the .NET Framework on the Exchange server let alone software which does not come from MS*). In this case, the push notifications work like this:
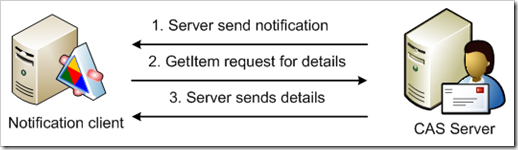
This makes three network hops to get the properties of a changed item. What makes things worse is the fact that the notifications are send for each modified element. With store event sinks, one could specify a filter so that the sink was only triggered for a subset of elements.
So, while store event sinks are certainly no unconfined pleasure to use, they are far more suited for certain scenarios. I would rather see them being replaced by a managed solution (like transport agents) than the stuff that is coming now.
By the way, the OnTimer, OnMdbShutdown, OnMdbStartup event sinks are being removed without any replacement.
Enough ranting for one day...
* Now, one could argue that a store event sink has far more impact on the Exchange server and those administrators would also hesitate to install them... while that is technically true, there is no other option yet, so they have to swallow that pill.
63e834db-504e-429a-bc32-fea68ee74f70|5|5.0